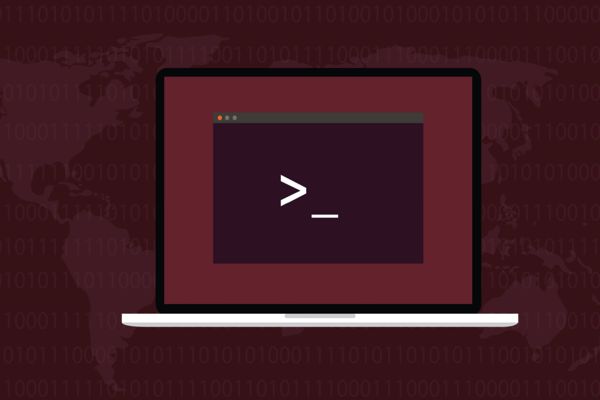
Mastering Bash Conditionals: 6 Examples for Better Scripting
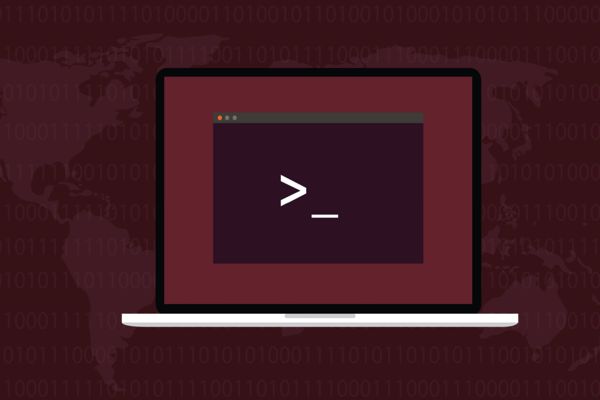
Learn how to make your Bash scripts more powerful with if statements Ask questions and run different code sections based on the answers Discover the different types of conditional tests and how to use nested if statements and the case for if
Conditional execution is essential in Bash scripting, and the if statement provides a way to run different sections of code based on the outcome of a decision. If statements are also known as if then statements or if then else statements. If the condition is true, the script executes a specific code, and if the condition is false, the script executes a different code. The decision can be based on various factors, such as the value of a variable, the presence of a file, or whether two strings match. Without conditional execution, the script's capabilities are limited, and it cannot produce practical solutions to real-world problems. The if statement is the most commonly used means of achieving conditional execution, and the simplest form of the if statement is as follows:
If the specified condition is true, the statements within the then clause are executed. Alternatively, you may come across an if statement in scripts written by others that includes a semicolon and the keyword "then" after the condition, followed by the statements to be executed. It is important to keep in mind that the if statement must always be concluded with the keyword "fi".
Ensure that there is a space between the first bracket "[ " and the second bracket " ]" in the conditional test. If you choose to place the then keyword on the same line as the conditional test, remember to use a semi-colon ";" after the test. You can include an optional else clause to execute code if the condition test proves false. The else clause does not require a then keyword. The following script provides a straightforward example of an if statement with an else clause. The conditional test verifies whether the customer's age is greater than or equal to 21. If so, the customer may enter the premises and the then clause is executed. If they are not old enough, the else clause is executed, and they are not allowed in.
Rewritten:
To run the script, copy the above code into a text editor and save it as "if-age.sh". Make sure to make the file executable by using the command "chmod +x if-age.sh". This script checks the age of a customer and allows them to enter if they are 21 or older.
Execute the script by running "./if-age.sh". Modify the customer_age variable to a value less than 21, for example, 18. Save your changes and run the script again. This time the condition will return false, and the else clause will be executed. The output will be displayed with the corresponding images and header.
The elif statement allows for additional conditional tests to be added, and as many elif clauses as needed can be used. Each one will be evaluated in order until one is found to be true. If none of the elif conditions are met, the else clause, if included, will execute. In the script provided, the program prompts the user to enter a number and then determines whether it is odd or even. Since zero is even, no further testing is necessary. For all other numbers, the modulo of dividing by two is calculated to determine if there is a remainder. If there is no remainder, the number is divisible by two and is therefore even.
After inputting a number, the script uses the "if-else" statement to determine whether it is an even or odd number. The message displayed to the user includes the number entered and whether it is even or odd. To execute the script, copy the code to an editor and save it as "if-even.sh", then make it executable using chmod. Running the script multiple times and checking the output confirms its functionality.
When using conditional tests in if statements, the brackets "[]" serve as a shorthand for calling the test program. This means that all the comparisons and tests supported by the test program can be used in if statements. Some examples of these tests include: checking if an expression is false with "! expression", checking if the length of a string is greater than zero with "-n string", checking if a string is empty with "-z string", checking if two strings are the same with "string1 = string2", checking if two strings are different with "string1 != string2", checking if two integers are equal with "integer1 -eq integer2", and checking if one integer is greater than another with "integer1 -qt integer2".
Different Forms of Conditional Test
Conditions can be checked in Bash using several flags such as "-lt" to check if an integer is less than another, "-d" to check if a directory exists, "-e" to check if a file exists, "-s" to check if a file exists and has a size greater than zero, "-r" to check if a file exists and has read permission, "-w" to check if a file exists and has write permission, and "-x" to check if a file exists and has execute permission. Both files and directories can be checked using relative or absolute paths. It's important to note that the equals sign and "-eq" are not the same; the former performs a character comparison while the latter performs a numerical comparison, as demonstrated using the test program in the command line.
When comparing strings or numbers in bash scripts, it is important to use the correct operator. In the case of comparing strings, using the equals sign " = " will give a false response if the strings are not exactly the same. However, if you want to compare numbers, it is important to use the correct numerical operator, such as "-eq", which will return a true response if the values are the same numerically. To use wildcard matching in a conditional test, the double bracket "[[ ]]" syntax can be used.
The script checks the current user's account name and prints it if it ends in "ve". If it doesn't end in "ve", the script notifies the user and ends. This can be achieved by nesting an if statement within another if statement.
Rewritten:
While it may be acceptable, nesting if statements can make code harder to read and maintain, especially when dealing with more than two or three levels. To improve code logic, consider reorganizing the script.
Take this example: a script that determines a shop's opening hours based on the current day. One to seven represents Monday to Sunday, respectively. The script reports that the shop is open on weekdays and Saturdays, but closed on Sundays. If open, it runs a nested if statement to check if it's Wednesday, and reports that the shop is only open in the morning.
#!/bin/bash
# Get the day as a number 1..7
day=$(date +"%u")
if [ $day -le 6 ]
then
## The shop is open
if [ $day -eq 3 ]
then
# Wednesday is half-day
echo "On Wednesdays we open in the morning only."
else
# regular week days and Saturday
echo "We're open all day."
fi
else
# not open on Sundays
When executed, the script will output "It's Sunday, we're closed." if the computer's clock is set to a Sunday. To try it out for yourself, copy the script into an editor, save it as a file called "if-shop.sh", and make it executable using the chmod command. While the if statement is a powerful tool for conditional execution in programming and scripting, it may not always be the best solution.